GORM ( ORM library for Golang) is a developer-friendly, full-featured ORM library for GoLang. As is true for everything Go, GORM comes with wonderful documentation (https://gorm.io/index.html) and a playground to test new ideas and functionalities online (https://github.com/go-gorm/playground). The easiest way to setup your own personal playground is to install docker-compose (https://docs.docker.com/compose/install/).
After completing the setups a developer is always interested in understanding what makes a library tick. Here are a few of our additional understandings that would probably tickle your brains as well.
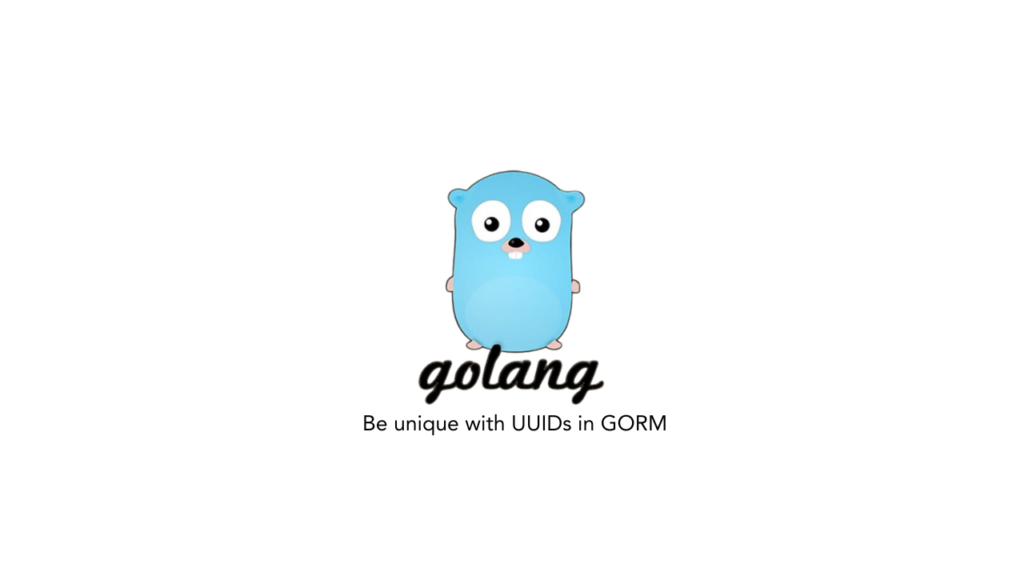
Creating UUIDs
You may want UUIDs for multiple reasons in your application, and using GORM it is easy to add an UUID generator to your code. Here is a gist of how to get it done:
Resolve dependencies:go get github.com/satori/go.uuid
go get github.com/jinzhu/gorm
Create a Base class to embed in your Models:type BaseUuid struct {
ID uuid.UUID gorm:"type:char(36);primary_key"
}
func (baseUuid *BaseUuid
) BeforeCreate(scope *gorm.Scope) error {
return scope.SetColumn("ID", uuid.NewV4())
}
You can now easily embed the BaseUuid struct in your Models just like a gorm.Model struct.
Access Dockerized Databases
The GORM playground provides development and testing across four different databases.
While it is easy to retrieve data and display the values on console, we might also be inclined to directly access these databases and validate our logic directly. You are invited to continue reading our pevious article on Dockerized Databases to understand how this can be achieved.
Medium: Dockerized Databases
In the end, we would like to acknowledge contribution of Renee French for Gopher images.
Your comments are most welcome.
You may like to explore other wonderful tutorials on MongoDB, Python etc. on this website. We have a YouTube channel covering all the latest technology changes.
MongoDB Replication on multiple AWS EC2 instances : Step by Step Guide
How to quickly create a cluster in MongoDB Atlas | MongoDB Atlas Tutorial
Happy Learning!