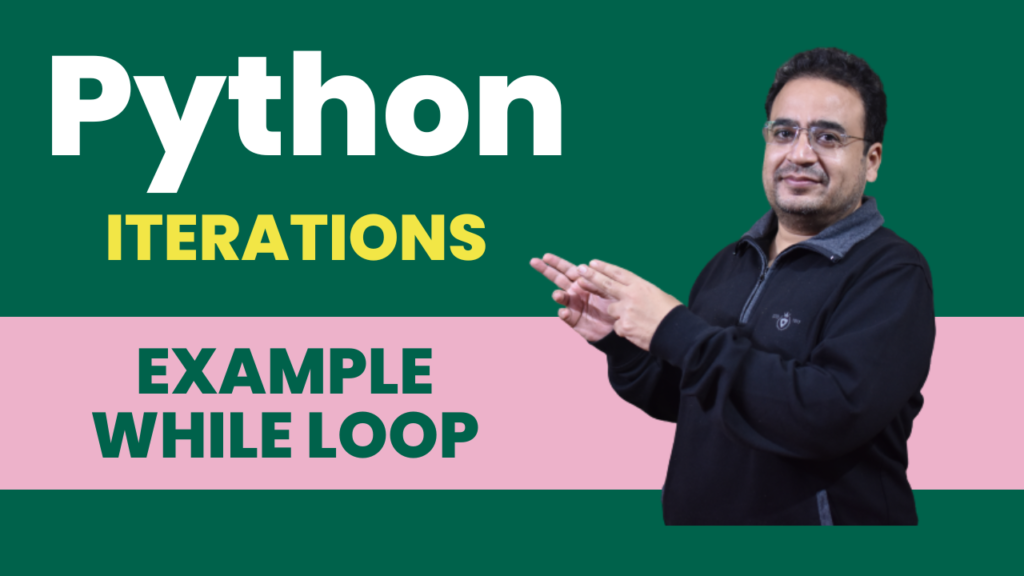
Iteration is a key concept and it represents to do a specific task repeatedly. Iterations can be executed based on certain conditions. We can implement iterations in multiple ways in Python i.e. using for loop, while loop etc. (discussed in separate sections).
In this post, I’ve used Python iteration concept using Python while loop over Python list. The post is structured as brief description of problem statement followed by a formula and then Python implementation.
Problem statement: Suppose you deposit $1,000 into a savings account and let it accumulate at 5% interest compounded annually. Write a function to determine how many years would you take to accumulate $10,000. Return number of years.
Compound Interest Formula:
Interest = Principal * (1 + ( Rate_Of_Interest/ 100))years
Where:
Principal = Amount initially
Rate_Of_Interest = Rate of Interest at an annual level.
years = Number of years, for which the compound interest needs to be calculated
Python Implementation
depost_amt = 1000
roi = 5
years = 0
final_amt = 0
while( final_amt <= 10000):
years+= 1
final_amt = depost_amt * pow((1+(roi/100)), years)
print(final_amt, years)
#python #pythoniterations #pythonwhile #pythonexample #learnwithneeraj #learnpythonwithneeraj
Other Python problems:
Learn MongoDB with my following detailed video series
AWS learning resources over my YouTube channel.